π Solving 'Top K Frequent Elements' Using Bucket Sort
tl;dr: An efficient approach to finding the k most frequent elements in an array
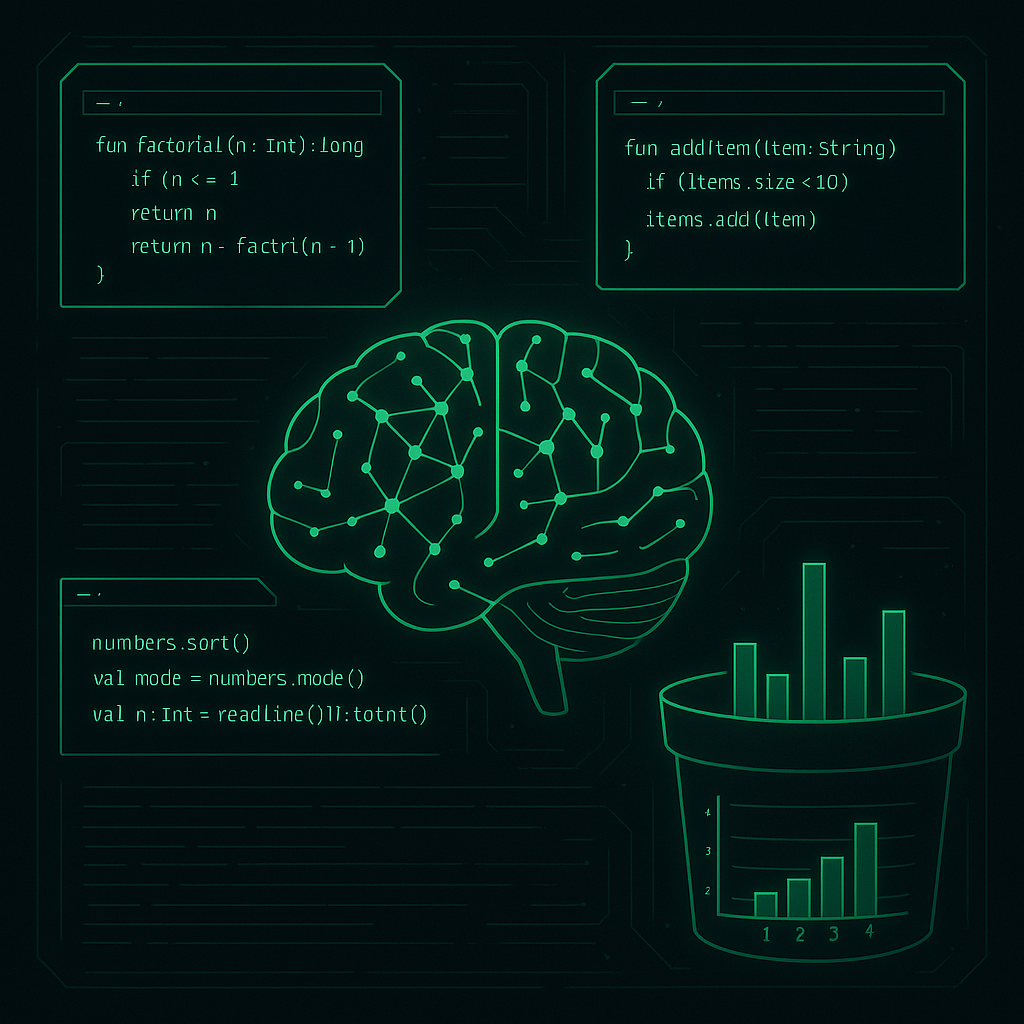
π Problem Statement
The problem Top K Frequent Elements requires us to return the k
most frequent elements from an array of integers.
π‘ My Initial Thought Process
At first, I thought of using a HashMap to count the frequency of each element, and then iterating through all keys to find the top k
elements.
But then I hit a roadblock:
- How do I efficiently find the top
k
frequent elements? - If I just iterate through the map
k
times, I end up with an O(NΒ²) solution in the worst case. - Sorting all elements by frequency would take O(N log N), which feels unnecessary when I only need the top
k
.
This forced me to rethink the problem.
π€― My Intuition & βAha!β Moment
Instead of focusing on sorting elements, I started thinking:
- βWhat if I group numbers by how frequently they appear?"
- "Since the maximum frequency an element can have is
N
, can I somehow use that?β
Then it clicked:
- I could create buckets where index = frequency.
- The most frequent numbers would naturally end up in the highest index buckets, and I could just iterate from the back to grab the top
k
. - This way, I avoid sorting entirely, keeping it O(N) time! π―
π My Optimized Approach: Bucket Sort
πΉ Steps to Solve Using Bucket Sort
- Count frequencies of each number using a
HashMap<Int, Int>
. - Group numbers by frequency in an array (
countArray
), whereindex = frequency
. - Collect the top
k
elements by iterating from the highest frequency bucket.
π» Code Implementation (Kotlin)
β³ Time Complexity Analysis
- Building freqMap β O(N)
- Filling countArray β O(N)
- Collecting top k elements β O(N) (worst case, when k β N)
- Overall Complexity: O(N) π (Fast & Efficient!)
π₯ What I Learned from This Struggle
- β Sometimes sorting isnβt necessaryβgrouping data in a structured way can be more efficient.
- β Thinking in terms of frequency instead of raw values led me to an intuitive solution.
- β Iterating from the highest frequency made it easy to grab the most frequent elements.
This problem really forced me to rethink how I approach optimization, and I loved that moment when the bucket idea clicked! π―
Letβs embark on this coding journey together! If you have any questions or want to discuss other approaches to this problem, feel free to reach out.