Introduction to DSA in Kotlin
tl;dr: Following my journey of learning DSA in Kotlin
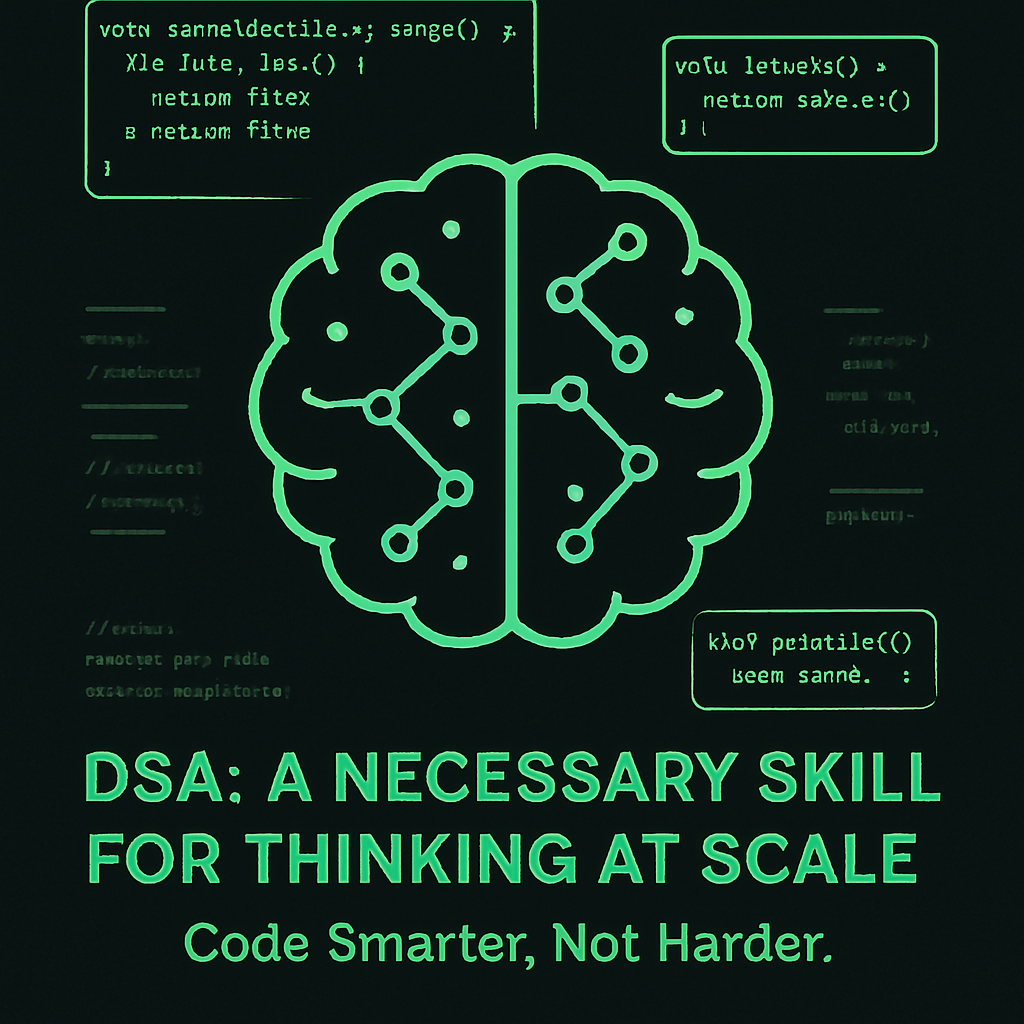
Welcome to Think in Code
This is the first post in our new “Think in Code” series, where I’ll be documenting what I’m learning in Kotlin. This will be a collection of random topics as I explore the language and improve my coding skills.
Why Kotlin?
Kotlin is a modern programming language that offers many advantages:
- Concise and expressive - Reduce boilerplate code and write more readable solutions
- Fully interoperable with Java - Use existing Java libraries and frameworks seamlessly
- Safe by design - Avoid null pointer exceptions and other common pitfalls
I’ll be sharing my learning journey with no specific structure or promises - just daily explorations to become better at coding. All code examples in this series will be exclusively in Kotlin or Java.
fun main() {
// A simple example of using a list in Kotlin
val numbers = listOf(1, 2, 3, 4, 5)
val sum = numbers.sum()
println("The sum is $sum") // Output: The sum is 15
}
Let’s embark on this Kotlin learning journey together!
I’d like to thank my friend Shangeeth Sivan for being my accountability partner and motivating me to start this journey.